In the world of data structures and algorithms, the Stack is one of the fundamental concepts that every programmer should understand. It is widely used in various applications, from managing function calls to evaluating mathematical expressions. In this article, we’ll explore the Stack data structure, its core operations, and how to implement it using Java. Whether you’re a beginner or just looking to refresh your memory, this guide will provide you with a solid understanding of Stacks.
What is a Stack?
A stack is a linear data structure that operates on the Last In, First Out (LIFO) concept. This means that the last element added to the stack is the first one to be moved out. Think of a stack as a stack of plates—when you add a new plate, you place it on the top, and when you remove a plate, you take it from the top.
Key Concepts of Stack
- LIFO (Last In, First Out): The most important feature of a stack is that the element that is inserted last will be removed first.
- Top: Refers to the element that is currently at the top of the stack.
- Size: Represents the number of elements currently in the stack.
- Overflow: Occurs when trying to add an element to a full stack.
- Underflow: Occurs when trying to remove an element from an empty stack.
Stack Operations
Here are the core operations associated with a stack:
- Push: Adds an element to the top of the stack.

- Pop: Removes and returns the top element of the stack.
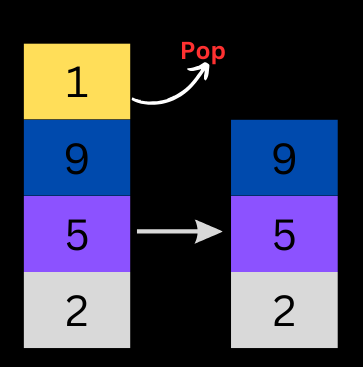
- Peek/Top: Returns the top element without removing it from the stack.
- isEmpty: Checks if the stack is empty.
- isFull: Checks if the stack is full (useful when using an array-based stack).
Example Operations
- Push: Inserting 10, 20, 30 into a stack results in
[10, 20, 30]
(30 being at the top). - Pop: Removing the top element would result in
[10, 20]
, with 20 now at the top. - Peek: This operation would return
30
without altering the stack.
Real-Life Examples of Stacks
Understanding how stacks work can be easier with a few real-world analogies:
- Browser History: When you navigate through web pages, each page you visit gets pushed onto a stack. Clicking the “back” button pops the most recent page, taking you to the previous one.
- Undo Mechanism: In text editors, each change is stored in a stack. When you undo an action, the latest change is popped from the stack.
- Call Stack in Programming: When a function calls another function, the calling function is paused until the called function is completed. This mechanism is managed using a stack.
Implementing a Stack in Java
Let’s look at how to implement a stack using Java. We’ll create a stack using arrays and methods for push
, pop
, peek
, and isEmpty
.
class Stack {
private int maxSize;
private int[] stackArray;
private int top;
public Stack(int size) {
maxSize = size;
stackArray = new int[maxSize];
top = -1; // stack is initially empty
}
public void push(int value) {
if (isFull()) {
System.out.println("Stack Overflow! Unable to push " + value);
} else {
stackArray[++top] = value;
}
}
public int pop() {
if (isEmpty()) {
System.out.println("Stack Underflow! No element to pop.");
return -1;
} else {
return stackArray[top--];
}
}
public int peek() {
if (isEmpty()) {
System.out.println("Stack is empty.");
return -1;
} else {
return stackArray[top];
}
}
public boolean isEmpty() {
return (top == -1);
}
public boolean isFull() {
return (top == maxSize - 1);
}
public static void main(String[] args) {
Stack myStack = new Stack(5);
myStack.push(10);
myStack.push(20);
myStack.push(30);
System.out.println("Top element is: " + myStack.peek());
myStack.pop();
System.out.println("Top element after pop: " + myStack.peek());
}
}
Explanation of the Code
- Constructor: Initializes the stack with a given size and sets the top pointer to
-1
. - push(): Adds an element to the stack after checking for overflow.
- pop(): Removes the top element if the stack is not empty.
- peek(): Returns the top element without modifying the stack.
- isEmpty() and isFull(): Check the stack’s status.
This simple implementation of a stack using arrays demonstrates how to manage data with LIFO ordering.
Applications of Stack
Stacks are widely used in both computer science and software development. Here are some key applications:
- Expression Evaluation: Stacks are used in evaluating arithmetic expressions, especially in converting infix to postfix notation.
- Parenthesis Checking: Balancing symbols like parentheses, brackets, and braces is achieved using stacks.
- Function Call Management: Modern programming languages use stacks to manage function calls and recursion.
- Backtracking: Algorithms like depth-first search (DFS) use stacks to backtrack and find paths in a graph or tree.
- Memory Management: Stack memory is used to store variables and function call information during execution.
Advantages of Using Stack
- Easy to Implement: Stacks are straightforward and easy to implement using arrays or linked lists.
- Memory Management: The stack memory structure is used for memory management in programming languages.
- Efficient Operations: Push and pop operations are highly efficient, with a time complexity of O(1).
Disadvantages of Using Stack
- Limited Size: If implemented using arrays, the stack size is fixed, leading to potential overflow.
- Lack of Random Access: Stacks do not allow direct access to elements other than the top element.
Conclusion
The Stack is an essential data structure in programming that is both simple to understand and powerful in its applications. Its LIFO nature makes it ideal for tasks like managing function calls, evaluating expressions, and implementing undo mechanisms. By mastering stacks, you can tackle a wide range of coding challenges more efficiently.
If you’re preparing for interviews or coding assessments, make sure to practice implementing and using stacks. Understanding the nuances of this data structure can give you a solid foundation in problem-solving.
Feel free to share your thoughts or any questions you have about Stacks in the comments below!